標籤:檔案 中文 3.0 das 學習筆記 top from ddl 參考
楊韜的Python/Jupyter學習筆記
- Python文法學習 https://zhuanlan.zhihu.com/p/24162430
Python 安裝庫安裝Jupyter Notebook
- 先安裝Python
- cmd 進入K:\Jupyter Notebook Python\Python_3.6.4\Scripts目錄
- cmd 輸入 pip install jupyter 開始安裝
- 運行 Python_3.6.4\Scripts下的 jupyter-notebook.exe
安裝numpy 數學包
到https://pypi.python.org/pypi/numpy#downloads 下載對應版本的numpy-1.14.0-cp36-none-win_amd64.whl 檔案,複製到Scripts目錄下安裝
cmd運行 在K:\Jupyter Notebook Python\Python_3.6.4\Scripts>
pip install numpy-1.14.0-cp36-none-win_amd64.whl
安裝繪圖包matplotlib
- cmd 輸入 pip install matplotlib
安裝pandas 資料輸入輸出庫
- cmd 輸入 pip install pandas
安裝seaborn繪圖庫
- cmd 輸入 pip install seaborn
pip安裝命令
- 安裝 pip install jupyterthemes
- 安裝最新版本 pip install --upgrade jupyterthemes
安裝指定版本 pip install jupyterthemes==0.18.3
- 實際上pip instal 既可以安裝本地.whl 也可以線上安裝
若線上安裝失敗,如UnicodeDecodeError: ‘gbk‘ codec can‘t decode 這類問題。可能需要安裝之前的指定版本
import math
math.sin(3)
0.1411200080598672
words = ['cat', 'window', 'defenestrate']for w in words: print("單詞 "+w, "長度"+str(len(w)))
單詞 cat 長度3單詞 window 長度6單詞 defenestrate 長度12
# 繪圖包 matplotlib 的使用# https://liam0205.me/2014/09/11/matplotlib-tutorial-zh-cn/# http://codingpy.com/article/a-quick-intro-to-matplotlib/import matplotlib.pyplot as plt # 需要先包含繪圖包 import numpy as npx = np.arange(20)y = x**2plt.plot(x, y)plt.show() # 顯示圖形#參考 http://codingpy.com/article/a-quick-intro-to-matplotlib/
$$ \int_0^{+\infty} x^2 dx $$
# 自訂曲線的外觀x = np.linspace(0, 2 * np.pi, 50)plt.plot(x, np.sin(x), 'r-o', x, np.cos(x), 'g--') # r g 顏色 - 線型plt.show()
# 彩色映射散佈圖x = np.random.rand(1000)y = np.random.rand(1000)size = np.random.rand(1000) * 50colour = np.random.rand(1000)plt.scatter(x, y, size, colour) # 散佈圖plt.colorbar() #顏色欄plt.show()
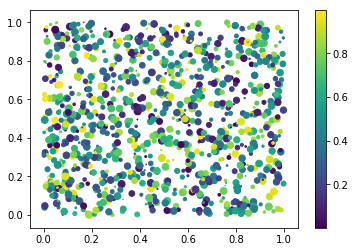
import matplotlib.pyplot as pltimport numpy as npfrom mpl_toolkits.mplot3d import Axes3Dnp.random.seed(42)# 採樣個數500n_samples = 500dim = 3# 先產生一組3維常態分佈資料,資料方向完全隨機samples = np.random.multivariate_normal( np.zeros(dim), np.eye(dim), n_samples)# 通過把每個樣本到原點距離和均勻分布吻合得到球體內均勻分布的樣本for i in range(samples.shape[0]): r = np.power(np.random.random(), 1.0/3.0) samples[i] *= r / np.linalg.norm(samples[i])upper_samples = []lower_samples = []for x, y, z in samples: # 3x+2y-z=1作為判別平面 if z > 3*x + 2*y - 1: upper_samples.append((x, y, z)) else: lower_samples.append((x, y, z))fig = plt.figure('3D scatter plot')ax = fig.add_subplot(111, projection='3d')uppers = np.array(upper_samples)lowers = np.array(lower_samples)# 用不同顏色不同形狀的表徵圖表示平面上下的樣本# 判別平面上半部分為紅色圓點,下半部分為綠色三角ax.scatter(uppers[:, 0], uppers[:, 1], uppers[:, 2], c='r', marker='o')ax.scatter(lowers[:, 0], lowers[:, 1], lowers[:, 2], c='g', marker='^')plt.show()# 參考 https://zhuanlan.zhihu.com/p/24309547
使用pandas資料輸入輸出庫
參考 http://python.jobbole.com/80853/
import pandas as pd df = pd.DataFrame({ 'A' : 1., 'B' : pd.Timestamp('20130102'), 'C' : pd.Series(1, index=list(range(4)), dtype='float32'), 'D' : pd.Series([1, 2, 1, 2], dtype='int32'), 'E' : pd.Categorical(["test", "train", "test", "train"]), 'F' : 'foo' })df
|
A |
B |
C |
D |
E |
F |
0 |
1.0 |
2013-01-02 |
1.0 |
1 |
test |
foo |
1 |
1.0 |
2013-01-02 |
1.0 |
2 |
train |
foo |
2 |
1.0 |
2013-01-02 |
1.0 |
1 |
test |
foo |
3 |
1.0 |
2013-01-02 |
1.0 |
2 |
train |
foo |
df.B
0 2013-01-021 2013-01-022 2013-01-023 2013-01-02Name: B, dtype: datetime64[ns]
使用Seaborn繪圖庫
Seaborn本質上使用Matplotlib作為核心庫,預設情況下就能建立賞心悅目的圖
# http://python.jobbole.com/80853/import seaborn as sns # Load one of the data sets that come with seaborntips = sns.load_dataset("tips") sns.jointplot("total_bill", "tip", tips, kind='reg');
tips.head()
|
total_bill |
tip |
sex |
smoker |
day |
time |
size |
0 |
16.99 |
1.01 |
Female |
No |
Sun |
Dinner |
2 |
1 |
10.34 |
1.66 |
Male |
No |
Sun |
Dinner |
3 |
2 |
21.01 |
3.50 |
Male |
No |
Sun |
Dinner |
3 |
3 |
23.68 |
3.31 |
Male |
No |
Sun |
Dinner |
2 |
4 |
24.59 |
3.61 |
Female |
No |
Sun |
Dinner |
4 |
sns.lmplot("total_bill", "tip", tips, col="smoker");
其它參考
http://blog.csdn.net/qq_34264472/article/details/53814653
https://www.cnblogs.com/kylinlin/p/5236601.html
http://blog.csdn.net/u013082989/article/details/73278458
https://www.cnblogs.com/gczr/p/6767175.html
Python·Jupyter Notebook各種使用方法記錄
http://blog.csdn.net/tina_ttl/article/details/51031113
Jupyter更換主題
https://github.com/dunovank/jupyter-themes
安裝主題
- pip install jupyterthemes
若出現UnicodeDecodeError: ‘gbk‘ 中文問題,可安裝之前的版本
pip install jupyterthemes==0.18.3
查看已安裝帶主題
- 命令列 jt -l
應用主題
- 命令列 jt -t onedork -f fira -fs 13
-fs 13 字型
回複原始狀態
- jt -r
# 嵌入視頻from IPython.display import VimeoVideoVimeoVideo("63250251",with=600, height=400)
File "<ipython-input-21-5e0fd48278da>", line 3 VimeoVideo("63250251",with=600, height=400) ^SyntaxError: invalid syntax
# 互動圖表import ipywidgetsa=ipywidgets.IntSlider(value=5,min=0, max=10, step=1)a
ipywidgets.Text()
# 嵌入HTML頁面from IPython.display import HTMLHTML('<iframe src="https://www.baidu.com" width=800 height=400></iframe>')
# 運行外部.py程式# %run xxxx/xxxx.py
# Geo-Json
安裝jupyter lab Python IDE
- pip install jupyterlab
執行 jupyter lab
楊韜的Python/Jupyter學習筆記