[TOC]
Inherit the basic knowledge definition:
Inheritance is an important means for reuse . By inheriting a class, inheritance is the modeling of relationships between types, the sharing of common things, and the realization of something different in their nature.
Inheritance Relationship:
Changes in the access relationships of base class members in derived classes under three inheritance relationships (figure)
Raise a chestnut (public succession)
"' C + +
Class Person
{
Public:
Person (const string& name)
: _name (name)
{}
void Display ()
{
Cout<<_name <<endl;
}
Protected:
string _name; Name
String _sex;
};
Class Student:public person//Public inheritance
{
Protected:
int _num; School Number
};
### 继承图例解释: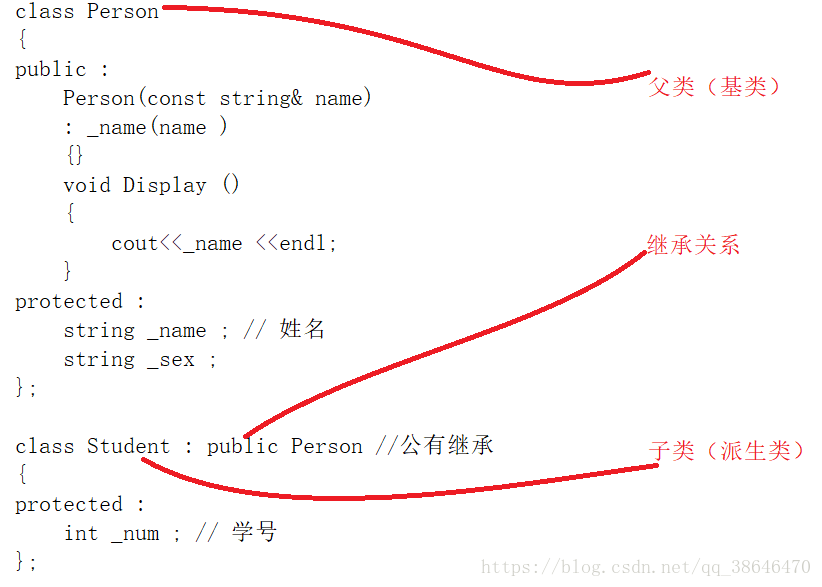**私有继承和保护继承很少用到,我们重点要掌握公有继承**### 继承与转换--赋值兼容规则--public继承1. 子类对象可以赋值给父类对象(切割/切片)2. 父类对象不能赋值给子类对象3. 父类的指针/引用可以指向子类对象4. 子类的指针/引用不能指向父类对象(可以通过强制类型转换完成)```C++class Person{public: void Display() { cout << "AA" << endl; }protected: string _name; // 姓名};class Student : public Person{public: int _num; // 学号};int main(){ Person a; Student b; a = b; //子类对象赋值给基类对象(切片)这个特性是编译器支持的 b = a; //父类对象不能赋值给子类对象 Person *p1 = &b; //特性3 //Person &a1 = b; //特性3 Student *p2 = (Student*)&a; //特性4 Student& b1 = (Student&)a; //特性4 getchar(); return 0;}
Scopes in the inheritance system
- In the inheritance system, both the base class and the derived class have separate scopes.
- A subclass member (member function, member variable) with the same name in the child class and parent class will mask the parent class's direct access to the member. (in subclass member functions, you can use the base class:: base class member access)--hide (redefine)
- = = Note In practice it is best not to define a member of the same name = = in the inheritance system.
class Person{public:Person(const char *name = "",int num = 0) :_name(name) ,_num(num){}
Protected
string _name; Name
int _num;
};
Class Student:public Person
{
Public
Student (const char* name = "", const int NUM1 = 0, int num2 = 0)
:P Erson (NAME,NUM1)
, _num (num2)
{}
void Display ()
{
cout << _num << Endl;
cout <<person:: _num << endl;//must show the base class scope to print base class members
}
Protected
int _num; School Number
};
int main ()
{
Person A ("Boday", 15);
Student B ("Crash", 1502,17);
B.display ();
return 0;
}
* * Operation Result: **! [Write a description of the picture here] (https://img-blog.csdn.net/20180422193345134?watermark/2/text/aHR0cHM6Ly9ibG9nLmNzZG4ubmV0L3FxXzM4NjQ2NDcw/ FONT/5A6L5L2T/FONTSIZE/400/FILL/I0JBQKFCMA==/DISSOLVE/70) It is clear that the members of the subclass are printed, and the members of the parent class are hidden, ("This is the Hidden") # # # Default member functions for derived classes & #8195; In an inheritance relationship, if the six member functions are not displayed in a derived class, the compilation system will default to the six default member functions. [Write a description of the picture here] (https://img-blog.csdn.net/20180421222558568?watermark/2/text/aHR0cHM6Ly9ibG9nLmNzZG4ubmV0L3FxXzM4NjQ2NDcw/ FONT/5A6L5L2T/FONTSIZE/400/FILL/I0JBQKFCMA==/DISSOLVE/70) * * to a chestnut say the first four of the default member functions (' The last two are not used ') * * ' CppClass person{ Public:person (const char *name = "", int num = 0)//Parent class constructor: _name (name), _num (num) {} ~person ()//Parent class destructor {cout << "~person ()" << Endl; } person (const person& p)//Parent copy constructor: _name (P._name), _num (p._num) {} person& operator= (cons T person& p)//Parent class assignment operator overload {if (this = &p) {_name = P._name; _num = P._num; } return *this; }protected: string _name; name int _num;}; Class Student:public Person{public:student (const char* name = "", const int NUM1 = 0, int num2 = 0)//Sub-class constructor: Person (NAME,NUM1), _num (num2) {} ~student ()//Sub-class destructor {cout << "~student ()" << Endl; } Student (const student& s)//Subclass copy Constructor:P Erson (s), _num (s._num) {} student& operator= (con St student& s)//subclass assignment operator overload {person::operator= (s);//Display Call parent class assignment operator overload _num = S._num; }protected:int _num; Study number};
先调用父类构造函数,在调用基类构造函数;析构函数调用顺序与构造函数相反(先构造后析构,这个和栈的规则有关(先入后出))
Inheritance mode (single inheritance, multiple inheritance, diamond inheritance)
1. Single-Inheritance definition: When a subclass has only one direct parent, the inheritance relationship is referred to as a single-Inheritance code example:
class A{protected: int _a;};class B : public A //B类 继承 A类{protected: int _b;};
2. Multiple inheritance definitions: One subclass has two or more
直接父类
This inheritance relationship is called a multiple-inheritance code example:
class A{protected: int _a;};class B {protected: int _b;};class C : public A,B{protected: int _c;};
3. Diamond Inheritance code example:
class Person{public: string _name; // 姓名};class Student : public Person{protected: int _num; //学号};class Teacher : public Person{protected: int _id; // 职工编号};class Assistant : public Student, public Teacher{protected: string _majorCourse; // 主修课程};void Test(){ // 显示指定访问哪个父类的成员(二义性问题) Assistant a; a.Student::_name = "xxx"; a.Teacher::_name = "yyy";//数据冗余问题}
It is obvious that there are problems in diamond inheritance, and there are ambiguity and data redundancy problems. In order to solve this problem, virtual inheritance is introduced.
Virtual Inheritance: Solving the two semantics of diamond inheritance and the problem of data redundancy
When declaring a derived class, specifies how the inheritance is declared as virtual inheritance. Such as
class A{public: int _a;};class B : virtual public A //声明为虚基类{protected: int _b;};class C : virtual public A //声明为虚基类{protected: int _c;};class D : public B,public C{protected: int _d;};
Look at the test results:
void Test(){ D d; d._a = 10;}
Is not very confused how to solve the problem? that's going to go deep into the bottom.
Here in the virtual inheritance with a virtual base table to hold the offset, so B and C class using a virtual base table to hold a relative to B and C offset, when the virtual inheritance of a will be stored in a common area, which is a good solution to the two of the semantic problem, but also save space.
Virtual inheritance is a good solution to the problems caused by diamond inheritance.
It is recommended that you write down the code to debug, while looking at memory changes.
An explanation of inheritance in C + +