Goal
- function parameter and return value function
- The return value of the function is advanced
- Parameters of the function step by step
- Recursive functions
01, function parameter and return value function
The function can be combined according to whether there are parameters and There are no return values , altogether 4 kinds of combinations
1, no parameter, no return value
2, no parameter, with return value
3, with parameters, no return value
4, with parameters, with return values
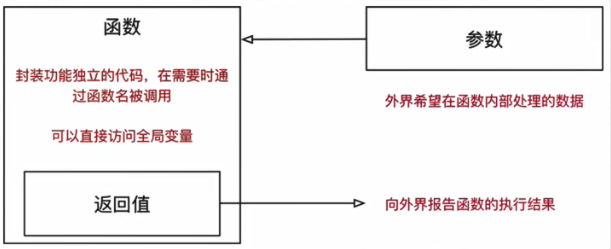
when defining a function , whether to receive parameters, or whether to return results , is determined according to the actual functional requirements .
1, if the function internal processing of data is not determined , you can transfer the external data parameters inside the function
2, you can increase the function return value if you want to report execution results to the outside after a function is executed.
02, the return value of the function is advanced
- In program development, it is sometimes desirable to tell a call to this result after a function is executed so that the caller can follow up on the specific result
- The return value is the result of a function that finishes work and finally gives the caller
- Use
return
key values in functions to return results
- Call the function side, you can use the variable to receive The return result of the function
question : Can I return multiple results after a function is executed?
Example-temperature and humidity measurements
- Suppose you want to develop a function that can return the current temperature and humidity at the same time
- The function of returning temperature first is as follows:
def measuring(): """测量温度和湿度""" print("测量开始 ----") temp = 23 humidity = 35 print("测量结束 ----") # 元组 - 可以包含多个数据,因此可以使用元组让函数一次返回多个值 # 如果函数返回的类型是元组,小括号可以省略 # return (temp, humidity) return temp, humidity # 元组 result = measuring() print(result) # 需要单独的处理温度或者湿度 print(result[0]) print(result[1]) # 如果函数返回的类型是元组,同时希望单独处理元组中的元素 # 可以使用多个变量,一次接收函数的返回值 # 注意,使用多个变量接收结果时,变量的个数应该和元组中元素的个数保持一致 t_temp, t_humidity = measuring() print("测量的温度为: %.2f" % t_temp) print("测量的湿度为: %.2f" % t_humidity) # 结果呈现 测量开始 ---- 测量结束 ---- (23, 35) 23 35 测量开始 ---- 测量结束 ---- 测量的温度为: 23.00 测量的湿度为: 35.00
Exchange two numbers
# 定义变量 a = 100 b = 50 # 解法一:使用其他变量 c = a a = b b = c # 解法二:不使用其他变量 a = a + b b = a - b a = a - b # 解法三: python专有 a, b = (b, a) # 提示:等号右边是一个元组,只是把小括号省略了 a, b = b, a print("a = %d" % a) print("b = %d" % b) # 结果呈现 a = 50 b = 100
03, parameters of the function 3.1 immutable and variable parameters
Question 1: Is it possible to use an assignment statement for a parameter inside a function that will affect the actual parametric passed when the function is called? --No!
- Whether the passed arguments are variable or immutable
- Whenever an assignment statement is used for a parameter , a reference to the local variable is modified inside the function without affecting the reference to the external variable
def demo(num, num_list): # 在函数内部,针对参数使用赋值语句,不会修改到外部的实参变量 num = 100 num_list = [4, 5, 6] print("函数内部代码 num = %d" % num) print("函数内部代码 num_list = %s" % (num_list)) gl_num = 66 gl_num_list = [1, 2, 3] demo(gl_num, gl_num_list) print("函数外部代码 gl_num = %d" % gl_num) print("函数外部代码 gl_num_list = %s" % (gl_num_list)) # 结果呈现 函数内部代码 num = 100 函数内部代码 num_list = [4, 5, 6] 函数外部代码 gl_num = 66 函数外部代码 gl_num_list = [1, 2, 3]
Issue 2: If the passed parameter is a mutable type , inside the function, the method modifies the contents of the data, and it affects the external data as well.
def demo(num_list): # 使用方法修改列表的内容 num_list.append(6) print("函数内部代码 %s" % num_list) gl_num_list = [1, 2, 3] demo(gl_num_list) print("函数外部代码 %s" % gl_num_list) # 结果呈现 函数内部代码 [1, 2, 3, 6] 函数外部代码 [1, 2, 3, 6]
+=
- In
python
, a list variable word +=
is essentially a method that executes a list variable extend
and does not modify the reference of the variable
def demo(num, num_list): # num = num + num num += num # 列表变量使用 += 不会做相加在赋值的操作! # num_list = num_list + num_list # 本质上是在调用列表的 extend 方法 num_list += num_list # num_list.extend(num_list) print("函数内部代码 num = %d" % num) print("函数内部代码 num_list = %s" % (num_list)) gl_num = 66 gl_num_list = [1, 2, 3] demo(gl_num, gl_num_list) print("函数外部代码 gl_num = %d" % gl_num) print("函数外部代码 gl_num_list = %s" % (gl_num_list)) # 结果呈现 函数内部代码 num = 132 函数内部代码 num_list = [1, 2, 3, 1, 2, 3] 函数外部代码 gl_num = 66 函数外部代码 gl_num_list = [1, 2, 3, 1, 2, 3]
3.2 Default Parameters
- When defining a function, you can specify a default value for a parameter , which is called the default parameter.
- When a function is called, if there is no value passed in to the default parameter , the default value of the parameter specified when defining the function is used inside the function
- function default parameter, which simplifies the invocation of a function by setting the common value to the default value of the parameter
- Example: How to sort a list
gl_list = [6, 3, 9] # 默认安装升序排序 gl_list.sort() print("升序排序 %s" % gl_list) # 如果需要降序排序,需要执行 reverse 参数 # reverse 参数就是一个缺省值,默认值为:reverse=False gl_list.sort(reverse=True) print("降序排序 %s" % gl_list) # 结果呈现 升序排序 [3, 6, 9] 降序排序 [9, 6, 3]
specifying function Default Parameters
- Use an assignment statement after the parameter to specify the default value for the parameter
def print_info(name, gender=True): """ :param name: 班上同学的姓名 :param gender: True 男生,False 女生 :return: """ gender_text = "男生" if not gender: gender_text = "女生" print("%s 是 %s" % (name, gender_text)) # 草帽海贼船上男生居多 # 提示:在指定缺省参数的默认值时,应该使用最常见的值作为默认值 print_info("蒙奇·D·路飞") print_info("罗罗诺亚·索隆") print_info("娜美", False) print_info("乌索普") print_info("文斯莫克·山治") print_info("托尼托尼·乔巴") print_info("妮可·罗宾", False) print_info("弗兰奇") print_info("布鲁克") print_info("甚平") # 结果呈现 蒙奇·D·路飞 是 男生 罗罗诺亚·索隆 是 男生 娜美 是 女生 乌索普 是 男生 文斯莫克·山治 是 男生 托尼托尼·乔巴 是 男生 妮可·罗宾 是 女生 弗兰奇 是 男生 布鲁克 是 男生 甚平 是 男生
Tips
1, the default parameter, you need to use the most common value as the default value!
2, if the value of a parameter cannot be determined , then the default value should not be set, the specific value is passed by the outside when the function is called!
Considerations for Default parameters
1) defined location of default parameters
- You must ensure that default parameters with defaults are at the end of the parameter list
- So, the following definitions are wrong!
def print_info(name, gender=True, title)
2) call a function with multiple default parameters
- when calling a function , if you have more than one default parameter, you need to specify the parameter name so that the interpreter can know the corresponding relationship of the parameter
def print_info(name,title="", gender=True): """ :param name: 班上同学的姓名 :param gender: True 男生,False 女生 :return: """ gender_text = "男生" if not gender: gender_text = "女生" print("[%s] %s 是 %s" % (title, name, gender_text)) # 草帽海贼船上男生居多 # 提示:在指定缺省参数的默认值时,应该使用最常见的值作为默认值 print_info("蒙奇·D·路飞") print_info("罗罗诺亚·索隆") print_info("娜美", gender=False) # 结果呈现 [] 蒙奇·D·路飞 是 男生 [] 罗罗诺亚·索隆 是 男生 [] 娜美 是 女生
3.3 Multi-valued parameters define functions that support multi-valued parameters
- Sometimes it may take a function to handle the number of arguments is indeterminate, this time, it is possible to use a multi-valued parameter
python
There are two kinds of multi-valued parameters
- Add a
*
tuple to receive before the parameter name
- Add two in front of the parameter name
*
to receive a dictionary
- Generally in the name of the multi-valued parameter, used to use a few two names
*args
--Store the tuple parameters, preceded by a*
**kwargs
--Store dictionary parameters, preceded by two**
args
is arguments
the abbreviation that has the meaning of the variable
kw
is keyword
the abbreviation that kwargs
can memorize the key value pair parameter
def demo(num, *nums, **person): print(num) print(nums) print(person) demo(1) # 结果呈现 1 () {}
def demo(num, *nums, **person): print(num) print(nums) print(person) demo(1, 2, 3, 4, 5, 6, name = "蒙奇·D·路飞", age = 18) # 结果呈现 1 (2, 3, 4, 5, 6) {‘name‘: ‘蒙奇·D·路飞‘, ‘age‘: 18}
hint : The application of multi-valued parameters will often appear in the framework developed on the network, know the multi-valued parameters, so that we can read the code of Daniel
Multi-valued parameter-calculates the and of any number of numbers
Demand
1, define a function sum_numbers
that can receive any number of arguments
2, functional requirements: Add all the passed numbers and return the cumulative result
def sum_numbers(*args): num = 0 print(args) # 循环遍历 for i in args: num += i return num result = sum_numbers(1, 2, 3, 4, 5) print(result) # 结果呈现 (1, 2, 3, 4, 5) 15
Unpacking of tuples and dictionaries
- When calling a function with a multivalued parameter, if you want to:
- A tuple variable is passed directly to the
args
- Pass a dictionary variable directly to the
kwargs
- You can use unpacking to simplify the transfer of parameters, and the way to split the package is:
- before the tuple variable, add a
*
- before the dictionary variable, increase the number of two
**
def demo(*agrs, **kwargs): print(agrs) print(kwargs) # 元组变量 、字典变量 gl_nums = (1, 2, 3) gl_dict = {"name" : "蒙奇·D·路飞", "age" : 18} # 拆包语法,简化元组变量和字典变量的传递 demo(*gl_nums, **gl_dict) demo(1, 2, 3, name = "蒙奇·D·路飞", age = 18) # 结果呈现 (1, 2, 3) {‘name‘: ‘蒙奇·D·路飞‘, ‘age‘: 18} (1, 2, 3) {‘name‘: ‘蒙奇·D·路飞‘, ‘age‘: 18}
04, Recursive function
The programming technique of the function call itself is called recursion.
4.1 Characteristic characteristics of recursive function
- A function internally calls itself
- Other functions can be called inside the function, but you can also call yourself inside the function
Code features
1, the code inside the function is the same, but different for the parameter , the result of processing is different
2, when a parameter satisfies a condition , the function no longer executes
- This is very important , usually referred to as a recursive exit, otherwise there will be a dead loop!
def sum_number(num): print(num) # 递归的出口,当参数满足某个条件时,不再执行函数 if num == 1: return # 函数自己调自己 sum_number(num - 1) sum_number(3) # 结果呈现 3 2 1
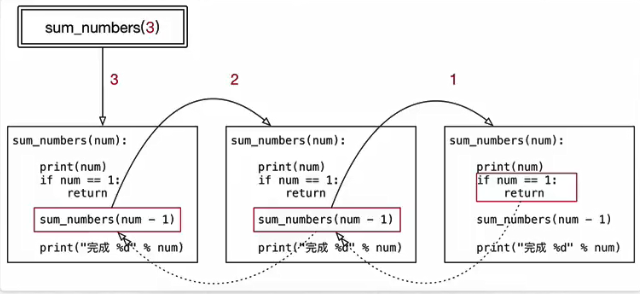
4.2 Recursive cases-Calculating cumulative numbers
Demand
1, define a functionsum_numbers
2, the ability to receive an num
integer parameter
3, calculate 1 + 2 + ... result of num
def sum_number(num): # 递归的出口,当参数满足某个条件时,不再执行函数 if num == 1: return 1 # 数字累加 num + (1 ...num -1) # 假设 sum_number 能够正确的处理 1 ...num -1 temp = sum_number(num - 1) # 两个数字相加 return num + temp result = sum_number(100) print(result) # 结果呈现 5050
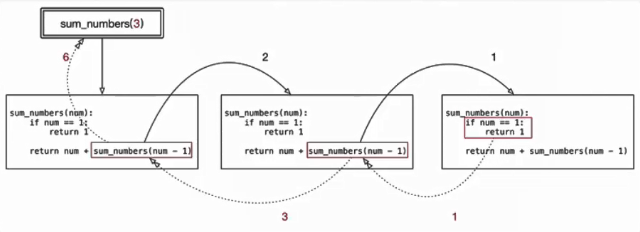
Tip : Recursion is a programming technique , and the first contact recursion will feel a little hard! Especially useful when dealing with uncertain cyclic body conditions , such as traversing the structure of the entire file directory
Python Advanced Variable Type---the step of the function