MyBatis Functional Architecture Design
Mage.png
Functional Architecture explained:
We divide MyBatis's functional architecture into three tiers:
(1) API Interface Layer: provides interface APIs for external use, where developers manipulate databases through these local APIs. Once the interface layer receives the call request, it invokes the data processing layer to complete the specific data processing.
(2) Data processing layer: Responsible for the specific SQL lookup, SQL parsing, SQL execution and execution result mapping processing and so on. Its primary purpose is to complete a database operation at the request of the call.
(3) Base support layer: Responsible for the most basic functional support, including connection management, transaction management, configuration loading and caching processing, these are common things, they are extracted as the most basic components. Provides the most basic support for the data processing layer of the upper layer.
Framework Architecture
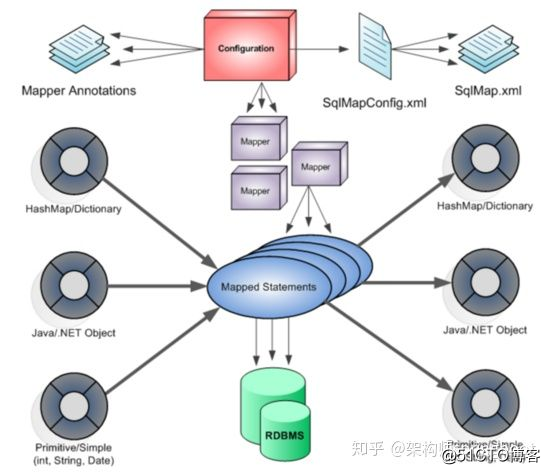
Frame Architecture explained:
This picture looks down from the top. MyBatis initialization, from the Mybatis-config.xml configuration file, parsing constructs the class, is the red box in the diagram.
(1) Load configuration: Configuration from two places, one is a configuration file, one is the Java code annotations, SQL configuration information is loaded into a Mappedstatement object (including the incoming parameter mapping configuration, executed SQL statement, result mapping configuration), stored in memory.
(2) SQL parsing: When the API interface layer receives a call request, it receives the ID of the incoming SQL and the incoming object (which can be a map, JavaBean, or base data type), and MyBatis finds the corresponding mappedstatement based on the SQL ID. Then the mappedstatement is parsed according to the incoming parameter object, and the SQL statements and parameters are finally executed.
(3) SQL execution: The final SQL and parameters are taken to the database for execution, resulting in the operation of the database.
(4) Result mapping: Transforms the results of the operational database into a mapped configuration that can be converted to HashMap, JavaBean, or basic data types and return the final result.
MyBatis Core Class
1, Sqlsessionfactorybuilder
The entrance to every MyBatis application is sqlsessionfactorybuilder.
It does this by creating a configuration object from an XML config file (which can, of course, be created yourself in the program), and then creating a Sqlsessionfactory object from the build method. It is not necessary to create a sqlsessionfactorybuilder every time you access mybatis, it is common practice to create a global object. The sample program is as follows:
private static SqlSessionFactoryBuilder sqlSessionFactoryBuilder;
private static SqlSessionFactory sqlSessionFactory;
private static void init() throws IOException {
String resource = "mybatis-config.xml";
Reader reader = Resources.getResourceAsReader(resource);
sqlSessionFactoryBuilder = new SqlSessionFactoryBuilder();
sqlSessionFactory = sqlSessionFactoryBuilder.build(reader);
}
org.apache.ibatis.session.Configuration is the core of mybatis initialization.
The configuration in Mybatis-config.xml, and finally parses the XML into a configuration class.
Sqlsessionfactorybuilder generates a configuration object based on the incoming data stream (XML), and then creates a default Sqlsessionfactory instance from the configuration object.
2. Sqlsessionfactory object created by Sqlsessionfactorybuilder:
Its main function is to create sqlsession objects, like Sqlsessionfactorybuilder objects, there is no need to create a sqlsessionfactory every time you access MyBatis, It is common practice to create a global object. A necessary property of the Sqlsessionfactory object is the configuration object, which is a config object that holds the MyBatis global configuration, typically created from an XML configuration file by Sqlsessionfactorybuilder. Here is a simple example:
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration PUBLIC
"-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<!--Configuration alias -->
<typeAliases>
<typeAlias type="org.iMybatis.abc.dao.UserDao" alias="UserDao" />
<typeAlias type="org.iMybatis.abc.dto.UserDto" alias="UserDto" />
</typeAliases>
<!-- Configuring environment variables -->
<environments default="development">
<environment id="development">
<transactionManager type="JDBC" />
<dataSource type="POOLED">
<property name="driver" value="com.mysql.jdbc.Driver" />
<property name="url" value="jdbc:mysql://127.0.0.1:3306/iMybatis?characterEncoding=GBK" />
<property name="username" value="iMybatis" />
<property name="password" value="iMybatis" />
</dataSource>
</environment>
</environments>
<!-- Configuring mappers -->
<mappers>
<mapper resource="org/iMybatis/abc/dao/UserDao.xml" />
</mappers>
</configuration>
3, Sqlsession
The main function of the Sqlsession object is to complete a database access and result mapping, which is similar to the session concept of the database, because it is not thread-safe, so the scope of the Sqlsession object needs to be restricted within the method. The default implementation class for Sqlsession is Defaultsqlsession, which has two properties that must be configured: Configuration and executor. The configuration text has been described here no more. Sqlsession the operation of the database is done through executor.
Sqlsession: Create defaultsqlsession By default and turn on first level cache, create executor, assign value.
Sqlsession has an important method Getmapper, as the name implies, is used to obtain mapper objects. What is a Mapper object? According to MyBatis's official manual, in addition to the initial and start MyBatis, the application needs to define some interfaces, which define the method of accessing the database, and the XML configuration file with the same name needs to be placed under the package path where the interface is stored.
The Getmapper method of sqlsession is to contact the application and MyBatis link, when the application accesses Getmapper, MyBatis generates a proxy object based on the type of interface passed in and the corresponding XML configuration file, which is called the Mapper object. Once the application obtains the Mapper object, it should access the MyBatis Sqlsession object through this mapper object, thus achieving the purpose of inserting into the mybatis process.
SqlSession session= sqlSessionFactory.openSession();
UserDao userDao = session.getMapper(UserDao.class);
UserDto user = new UserDto();
user.setUsername("iMybatis");
List<UserDto> users = userDao.queryUsers(user);
public interface UserDao {
public List<UserDto> queryUsers(UserDto user) throws Exception;
}
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="org.iMybatis.abc.dao.UserDao">
<select id="queryUsers" parameterType="UserDto" resultType="UserDto"
useCache="false">
<![CDATA[
select * from t_user t where t.username = #{username}
]]>
</select>
</mapper>
4, Executor
The Executor object is created when the configuration object is created and cached in the configuration object. The primary function of the Executor object is to call Statementhandler to access the database and store the query results in the cache (if the cache is configured).
5, Statementhandler
Statementhandler is where the database is actually accessed and calls Resultsethandler to process the query results.
6, Resultsethandler
Process the query results.
MyBatis member Levels & Responsibilities
Image.png
1, sqlsession as the main top-level API for MyBatis work, representing and database interaction sessions, complete the necessary database additions and deletions function
2, Executor MyBatis Actuator, is the core of MyBatis Dispatch, responsible for SQL statement generation and query cache maintenance
3. Statementhandler encapsulates the JDBC statement operation, which is responsible for the operation of Jdbcstatement, such as setting parameters, converting statement result set into a list collection.
4. Parameterhandler is responsible for converting the parameters passed by the user to the parameters required by the JDBC Statement.
5. Resultsethandler * is responsible for converting the resultset result set object returned by JDBC into a collection of list types;
6. Typehandler is responsible for mapping and conversion between Java data types and JDBC data types
7, Mappedstatement Mappedstatement maintained a <select|update|delete|insert> node of the seal
8. Sqlsource is responsible for dynamically generating SQL statements based on user-passed parameterobject, encapsulating information into Boundsql objects, and returning
9. Boundsql represents a dynamically generated SQL statement and corresponding parameter information
10, configuration MyBatis All the information is maintained in the Config object
MyBatis Architecture and principles